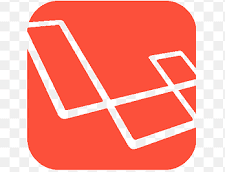
Ở phần 1 chúng ta đã xây dựng 1 ứng dụng đăng nhập bằng Nextjs. Phần 2 chúng ta sẽ tìm hiểu cách đăng nhập mạng xã hội cho các dự án Nextjs.
Tiếp theo ta vào file pages/login.tsx
để thêm button đăng nhập bằng Google.
import { useRouter } from "next/router";import React, { SyntheticEvent, useState } from "react";import Layout from "../layouts/Layout";const Login = () => {const [email, setEmail] = useState("");const [password, setPassword] = useState("");const router = useRouter();const submit = async (e: SyntheticEvent) => {e.preventDefault();await fetch("http://localhost:8000/api/login", {method: "POST",headers: { "Content-Type": "application/json" },body: JSON.stringify({email,password,}),}).then((res) => res.json()).then(async (data) => {localStorage.setItem("jwt_token_key", data.access_token);await router.push("/");});};return (<Layout><div className="login-page-wrapper"><form onSubmit={submit}><h1 className="h3 mb-3 fw-normal">Đăng nhập</h1><div className="form-floating"><inputtype="email"className="form-control"placeholder="Email"requiredonChange={(e) => setEmail(e.target.value)}/></div><div className="form-floating"><inputtype="password"className="form-control"placeholder="Mật khẩu"requiredonChange={(e) => setPassword(e.target.value)}/></div><button className="w-100 btn btn-lg btn-primary" type="submit">Đăng nhập</button></form><div className="social-login-wrapper"><button className="google">Đăng nhập bằng Google</button></div></div></Layout>);};export default Login;
Để thuận tiện cho việc xây dựng giao diện, chúng ta cần sử dụng sass để viết css dễ dàng và có nhiều tính năng hơn. Để cài đặt sass chúng ta chạy lệnh:
yarn add sass
Tiếp theo chúng ta thêm file login.scss
vào folder styles
với nội dung:
.login-page-wrapper {.social-login-wrapper {margin-top: 50px;.google {width: 100%;height: 48px;color: #fff;background: #e74c3c;border: 0;border-radius: 8px;font-size: 20px;}}}
Cuối cùng chúng ta sẽ import file login.scss
vào file _app.tsx
:
...import '../styles/login.scss'
Để tích hợp social login với Nextjs ta cần cài đặt package next-auth:
yarn add next-auth
Tiếp theo chúng ta sẽ thêm session
vào toàn bộ dư án bằng cách chỉnh sửa file _app.tsx
như sau:
// pages/_app.tsximport { SessionProvider } from "next-auth/react";import "../styles/globals.css";import "../styles/login.scss";function MyApp({ Component, pageProps: { session, ...pageProps } }) {return (<SessionProvider session={session}><Component {...pageProps} /></SessionProvider>);}export default MyApp;
Để thêm NextAuth.js
vào dự án chúng ta cần tạo file [...nextauth].tsx
bên trong thư mục pages/api/auth
:
// pages/api/auth/[...nextauth].tsximport NextAuth from "next-auth";import GoogleProvider from "next-auth/providers/google";export default NextAuth({providers: [GoogleProvider({clientId: process.env.GOOGLE_ID,clientSecret: process.env.GOOGLE_SECRET,}),],});
Chúng ta truy cập đường link https://console.cloud.google.com/ để tạo Google Login App.
Bước 1: Click vào My Project
để tạo Project
mới như hình
Bước 2: Click vào nút New Project
như hình
Bước 3 là đặt tên Project
và click nút Create
Bước 4: Click vào menu APIs & Services
> Enabled APIs & services
Bước 5: Config consent bằng cách click menu OAuth consent screen
và chọn Internal
hoặc External
sau đó nhấn CREATE
Bước 6: Nhập thông tin consent
Bước 7: Click menu Credentials
và tạo app Google Social Login bằng cách click vào nút CREATE CREDENTIALSCREDENTIALS
xong click tiếp vào OAuth client ID
Bước 8: Nhập thông tin và tạo app
Sau khi tạo xong, chúng ta sẽ có Client ID
và Client Secret
dùng để truy cập ứng dụng.
Để lưu thông tin cấu hình của dự án, chúng ta tạo file .env.local
ở thư mục gốc và lưu Client ID
và Client Secret
lấy được ở bên trên vào biến GOOGLE_ID
và GOOGLE_SECRET
như sau:
// .env.localGOOGLE_ID=247421812042-nk596tio7ds6rfermbf8tuj8sdpunmom.apps.googleusercontent.comGOOGLE_SECRET=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
Tiếp theo chúng ta xây dựng chức năng login Google bằng cách import thư viện next-auth/react
bên trong file pages/login.tsx
như sau:
// pages/login.tsx...import { signIn } from "next-auth/react";
Vào cập nhật function signIn vào button Đăng nhập bằng Google
// pages/login.tsx...<button className="google" onClick={() => signIn("google")}>Đăng nhập bằng Google</button>
Sau khi đã lấy được session khi login bằng google thì chúng ta sẽ chuyển user đến trang Homepage
// pages/login.tsx...const router = useRouter();useEffect(() => {(async () => {const session = await getSession();if (session) {router.push("/");}})();}, [router]);
Cuối cùng là cập nhật lại logic check login ở trang Homepage
// pages/index.tsx...useEffect(() => {(async () => {const session = await getSession();if (session) {setMessage(`Hi ${session.user.name}`);} else {const token = localStorage.getItem("jwt_token_key");try {const response = await fetch("http://localhost:8000/api/me", {headers: { Authorization: "Bearer " + token },});if (response.status !== 200) {localStorage.removeItem("jwt_token_key");await router.push("/login");} else {const content = await response.json();setMessage(`Hi ${content.data.name}`);}} catch (e) {localStorage.removeItem("jwt_token_key");await router.push("/login");}}})();}, [router]);
Vậy là chúng ta đã hoàn thành xong phần đăng nhập bằng google. Code tham khảo các bạn có thể xem tại đây: